Extend DatePipe To Show PST Time In Angular
Published on April 29th , 2021- Angular
- HTML
- CSS
- JS
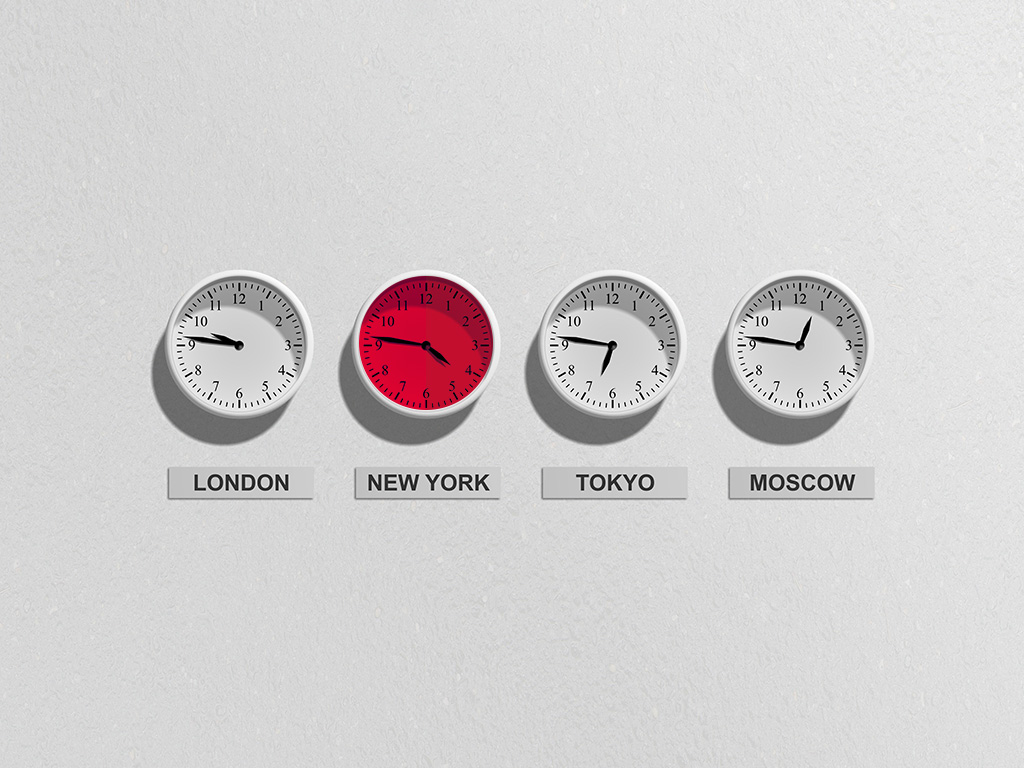
Recently I got a requirement to show UTC time as PST time. I know this could be easy peasy but I didn’t want to use moments nor I wanted to use some third-party library to transform it.
So I ended up transforming it manually using offset and since I only wanted to transform dates to PST timezone only, It was pretty easy to do so.
Here is the pipe I created to transform all UTC data that comes from the database to PST using offset.
import { Pipe, PipeTransform } from ‘@angular/core’;
import * as _moment from ‘moment-timezone’;
import { DatePipe } from ‘@angular/common’;
@Pipe({
name: ‘pstTime’
})
export class PstTimePipe extends DatePipe implements PipeTransform {
transform(date: any, format?: any): any {
let dateObj: Date = typeof date == ‘string’ ? new Date(date) : date;
let offset = 7 * 60 * 60 * 1000;
dateObj.setTime(dateObj.getTime() - offset);
return super.transform(dateObj, format, null, null) + ‘ PST’;
}
}
It will show the dates as Jul 31, 2019, 11:30 PM PST
which is exactly how I required.
Of course, You can extend this furthermore and make it more dynamic to support any timezones. But for now, this does it and I’m happy with it.
Thanks